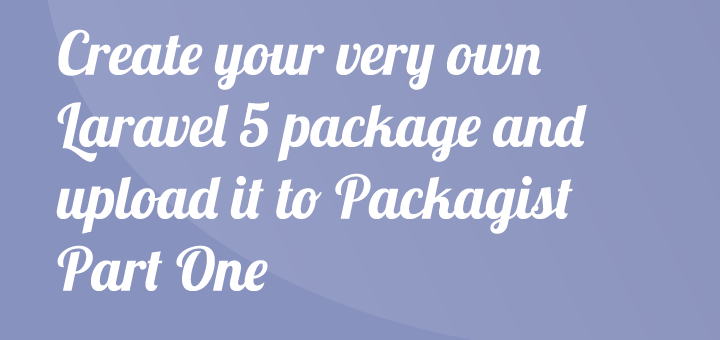
Create your own Laravel 5 package – Part 1
Laravel is where it’s all at right now, there are many different PHP Frameworks out there that you can use to speed up your coding and not needing to reinvent the wheel for every project you make. If we’re following the DRY (Don’t Repeat Yourself) principle we can create or use packages for common tasks we use a lot.
What are we making
We’re going to make a simple package that will return a user Gravatar or a link to the users profile, with the help of Facades we’ll be able to call it like Gravatar::method(). This is just a simple tutorial to get you started with your own package development.
Contents
Check out the finished package on GitHub
Create new Laravel 5 project
Let’s begin with a fresh copy of Laravel, I assume you have everything setup like composer and laravel global.
laravel new package-tutorial
When it has finished the installation we need to add our project into our homestead, open it up using homestead edit. And add the new project.
- map: package-tutorial.dev
to: /home/vagrant/Code/package-tutorial/public
When you’ve updated the homestead file you need to re-provision it with homestead provision, and add the new site into your hosts file. Great, now we have a clean install to work with! We will need a package called workbench, as I’m writing this you’ll need the dev-master version since it’s the only one updated for Laravel 5.
composer require xtwoend/workbench:dev-master --require-dev
You’ll also need to add it to your Service Providers in app/config/app.php.
Xtwoend\Workbench\WorkbenchServiceProvider::class,
Now we can see a new command if we run php artisan called workbench, we can using this create a new package. We’ll need to publish the config file for workbench so that we may setup our author credentials.
php artisan vendor:publish
We can find the config file at app/config/workbench.php and here you’ll enter your name and e-mail.
Create our Gravatar package
First off we’ll need to figure out what we’re going to name it, and the convention is to name the vendor as your lastname or site-name. I use jejje as my vendor name and we’re going to call the package gravatar, let’s generate the package with the workbench command.
php artisan workbench jejje/gravatar
Now we just need to wait for the dependencies to be pulled in, it won’t take long. When it’s finished you may find your newely generated package in /workbench/jejje/gravatar. You’ll find your package under the src folder, and a Service Provider has been generated called GravatarServiceProvider.php. We can now add this to our Service Provider list.
Oh, no! We’re getting an error message that the class is not found! I had to Google around to figure out why I was getting this error message, and the solution was to add this to the /bootstrap/autoload.php.
/*
|--------------------------------------------------------------------------
| Register The Workbench Loaders
|--------------------------------------------------------------------------
|
| The Laravel workbench provides a convenient place to develop packages
| when working locally. However we will need to load in the Composer
| auto-load files for the packages so that these can be used here.
|
*/
if (is_dir($workbench = __DIR__.'/../workbench'))
{
Xtwoend\Workbench\Starter::start($workbench);
}
Add facade and configuration file
We’ve now come so far that it is time to start coding and I think we should start with our Facade for easy access to our package methods like this Gravatar::method().
Create our Facade
In our package folder /workbench/jejje/gravatar/src/Jejje/Gravatar/ we will add a PHP class that extends the Laravel Facade, we’ll name it Gravatar.
<?php namespace Jejje\Gravatar;
use Illuminate\Support\Facades\Facade;
class Gravatar extends Facade {
/**
* Facade for our Gravatar Package
* so that we may use it like
* Gravatar::method();
*
* @return string
*/
protected static function getFacadeAccessor() {
return 'Jejje\Gravatar\Image';
}
}
The getFacadeAccessor binds the Facade to the PHP Class where all our logic will be stored and we’re going to name it Image, and we’ll do this in the next part of this tutorial since it’s going to be a long file with some nice methods. But just before we finish up I’d like to setup a configuration file that we’ll need in the main class.
Setup a Configuration file
It’s quite simple to make a configuration file where users can edit settings for our package, we’re not going to have many settings – we’re just doing it to get a light grasp on the concept. In our Gravatar folder create a folder called config and a file within it called config.php.
<?php
/* /workbench/jejje/gravatar/src/Jejje/Gravatar/config/config.php */
return [
'default_gravatar_size' => 100
];
s It’s a simple file that just returns an array as you can see, you can go as nuts as you like with settings and arrays within arrays if you need to. We need to register this in our GravatarServiceProvider.
<?php namespace Jejje\Gravatar;
use Illuminate\Support\ServiceProvider;
class GravatarServiceProvider extends ServiceProvider {
/**
* Indicates if loading of the provider is deferred.
*
* @var bool
*/
protected $defer = false;
/**
* Register the service provider.
*
* @return void
*/
public function register()
{
//
/**
* This will publish the config file when you do vendor:publish
*/
$this->publishes([
__DIR__.'/config/config.php' =>
config_path('jejje/gravatar/config.php'),
]);
}
/**
* Get the services provided by the provider.
*
* @return array
*/
public function provides()
{
return [];
}
}
When you have updated your Service Provider you should run vendor:publish so that the configuration file gets saved to your project config folder like this /config/jejje/gravatar/config.php. To finish up run;
php artisan vendor:publish
We’ve made great progress so far and I’ll see you in the next part of this series to add all our logic to make it work, and we’ll also look into how to publish our work.
Comments